INTRODUCTION:
Quadratic equations may be solved in four ways- by inspection; also known as by factoring, which does not work for every quadratic, by completing the square, by using the quadratic formula and by Newton-Raphson approximation method. Two methods of factorization have been frequently in use, factorization by grouping and factorization using factor theorem.
Factorization by grouping is applicable to quadratic expressions, while factorization using factor theorem has application that goes beyond polynomial of order 2. In this Paper, factorization of polynomials of order 2 and the subsequent solutions to their corresponding equations by determinant is proposed. The method is similar to the method of grouping in the sense that both employ two of the factors of the product of the two end-terms of the expression under consideration. The methods are however different in three respects.
Factorization by determinant makes use of the first three terms of the four terms, which are grouped during factorization by grouping in the factorization technique. Also factorization by determinant does not involve grouping exercise but involves a symmetric arrangement of the allowable pairs of the factors of the two end-terms of the equation, even with the last term not involved in sorting out the factors.
Lastly providing solution to polynomial equations of order 2 using this method can possibly take care of situations in which the solution is not exact and so can be seeing to be similar to Newton-Raphson approximation method in the sense of iteration. The method is advantageous over the method of grouping in two folds. It is computer oriented, and from the point of departure from the method of grouping, it embraces four operations; whereas method of grouping requires six operations considering manual approach.
The application program for the implementation of the algorithm can be generalized to accept user defined values for the coefficients of the first and the second power of the variable, and the constant term. Although the application/C++ program written is applicable to situations in which the equation has exact solutions, it may be extended to the case in which the equation has no exact roots and in this case, we will be dealing with real variables rather than integer variables and boundary condition can be imposed in the sub-problems of finding the l.c.f and/or h.c.f, as in divide and conquer approach, to solve such an equation.
THE ALGORITHM:
A real number has both positive and negative factors.
To factorize ax2 + bx + c by determinant, let f and g be the two factors of the product, +ac , of the coefficient, a, of x2 and the constant term, +c such that f + g = +b where +b is the coefficient of x. The operator is given by :
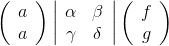
The determinant is defined here, as opposed to the common definition of determinant in relation to matrix, as

The objective is to determine the elements α, β, γ and δ, and they are as follow such that

The determinant is therefore given by:
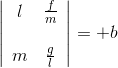
It is so called here because it resolves the factors of the said expression. The procedure is that 'l' and 'm' are found first, this is followed by the division of 'f' and 'g' by 'm' and 'l' respectively.
CASE 1: Case with positive a.
If a is positive, then l is the highest common factor (h.c.f) of a and g and m is the highest common factor of a and f.
CASE 2: Case with negative a.
If 'a' is negative, then l is the lowest common factor (l.c.f) of 'a' and 'g', while m is the highest common factor of a and f, OR l is the highest common factor of a and g, while m is the lowest common factor of a and f.
Hence there are two possible answers for this case. However, a complete factorization of both leads to the same result.
EXAMPLE: Obtain the factors of the polynomial below by 'determinant'

COMPUTER-BASED SOLUTION: - With a = -2, b = -1, c = +6.
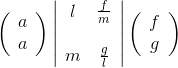
Where l = lcf(a,g),
m = hcf(a,f)
With the following definitions:

We write :
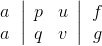
So, the solution of the equation

i.e, x = - u/l or x = - v/m
APPLICATION PROGRAM FOR IMPLEMENTING THE ALGORITHM
A C++ that determines the roots of a polynomial of order 2 by determinant.
#include<iostream>
using namespace std;
int main() {
int a, b, c, f, k, s, p, g, l, d, u, v;
cin >> a, b, c, f;
a = -2;
b = -1;
c = +6;
f = a*c;
cout << " enter the values of a, b and c: " "\n";
cout << " a = " << a << endl;
cout << " b = " << b << endl;
cout << " c = " << c << endl;
cout << " f = " << f << endl;
//determine the values of f and g
f = f; for ( f; f<=12; ++f) {
if ( f = 0 )
{ f = f + 1;
}
else
{ g = -12/f;
cin >> g;
p = g + f;
}
if ( p == b)
{
cout << " f = " << f << " , " " g = " << g << endl;
}
else f = f + 1;
continue;
}
//Determine the l.c.f of a and g
//
cin >> l;
l = -12;
for ( l = -12;l < 20; ++l )
{ if ( l = 0 )
{ l = l + 1;
}
else
{
d = g%l; k = a%l;
} if (d == 0 and k == 0 )
{ cout << " l.c.f of a and g = " << l << " \n";
}
else
continue;
}
/* determine the h.c.f of a and f */
int m, e, h;
cin >> m;
m = -20;
for ( m = -20; m < 40; ++m )
{
if ( m = 0 )
{ m = m + 1;
}
else
{ e = a%m; h = f%m;
} if ( e == 0 and h == 0 )
{
cout<< " h.c.f of a and f = " << m << " \n";
}
else
continue;
}
// write out the determinant
cin >> f, m, g, l;
u = f/m;
v = g/l;
cout << " ! " << l << "x" " " << u << " ! " << endl;
cout << " ! " " " " ! " << endl;
cout << " ! " " " " ! " << endl;
cout << " ! " " " " ! " << endl;
cout << " ! " " " " ! " << endl;
cout << " ! " << m << "x" " " << v << " ! " << endl;
//write out the expression in factor form
cout << " -2x2 - x + 6 = ";
cout << " ( " << l << "x" + u ;
cout << " ) ";
cout << " ( " << m << "x" + v ;
cout << " ) " << endl;
}
MANUAL-BASED SOLUTION: With a = -2, b = -1, c = +6
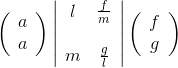
Since a = -2, b = -1 and c = +6, it follows that +ac = (-2)(+6) = -12, and
a is less than zero implies that l is the lowest common factor (l.c.f)
of a and g, while m is the
highest common factor of a and f, OR l is the highest common factor of a and g,
while m is the lowest common factor of a and f such that g + f = +b,
i.e g + f = -1, meaning that the allowable factors of +ac, -12,
that satisfy this are -4 and 3.
Hence l = -1, m = 2, g = 3 and f = -4.
The determinant is therefore given by:
The factors are therefore (-1x - 2) and (2x - 3) or (1x + 2) and (-2x + 3).
Hence the solution of the equation
= 0 is given by (-1x - 2)= 0 or 2x - 3 = 0,
giving x = -2 or x = 3/2, etc.
AREAS OF LIFE WHERE THE ALGORITHM MAY FIND APPLICATION:
It was the need to calculate land areas that motivated the Babylonians to discover the quadratic equation to begin with. Surface area is important in real estate, medicine, physics, and engineering. It affects how fast an object cools off (greater area equals quicker cooling), which is why machines that need to get rid of extra heat sometimes have little metal fins stuck on them to increase their surface area. It affects how quickly a droplet evaporates (greater area equals quicker evaporation).
It affects how quickly a chemical reaction proceeds (greater area equals quicker reaction). The fact that area is described by a quadratic equation and volume by a cubic equation affects many things in nature. Any object's surface area is proportional to x2—where x stands for how wide the object is—but its volume is proportional to x3. As you make the object bigger; that is, increase x, x3 will always grow faster than x2. This is why insects can't (lucky for us) grow to the size of dogs or whales: they breathe using surface area (x2) but their need for oxygen goes by body volume (x3). This is why elephants have fat legs: the strength of a leg-bone goes by cross-sectional area (x2), but the weight the bone has to bear goes by the volume of the elephant (x3) (Chris Budd and Chris Sangwin).
ACCELERATION
Quadratic equations are needed to predict the paths of accelerating objects. Acceleration is any change in speed. When the driver of a car steps on the gas or hits the brakes, the car accelerates or decelerates (goes faster or slower). When you drop a ball or throw it up in the air it accelerates, positive or negative. And almost any time a machine with moving parts is designed, from a CD player to a car engine to a jet plane, the people designing the product must deal with accelerations (Chris Budd and Chris Sangwin)
CAR TIRES
Car tires are made of rubber-like plastics derived from petroleum and interwoven with metal wires, and must work well despite thousands of miles of use, violent blows from bumps, fast turns, and other stresses. Your life depends on them every day, and their design is a complex art. Computer calculations are used to predict how a new tire design will behave, as this is much cheaper than casting actual tires in a trial-and-error way. One of the most important factors in modeling a tire using calculations is describing the mechanical properties of the "rubber" used in the tire: how it responds to stretching, squeezing, and twisting. In a class of new synthetic tire materials called "carbon black filled rubber compounds," it has been found that a cubic equation best describes the stress-strain relationship—that is, how much the material gives in response to a certain amount of force. This cubic equation is used in writing a computer program that will accurately predict how a tire made with these compounds will behave. (Chris Budd and Chris Sangwin)
JUST IN TIME MANUFACTURING Traditional economics treated supply and demand as the two factors deciding profitability in manufacturing. However, in the 1990s some Japanese manufacturers introduced a philosophy called "just in time" (JIT) manufacturing. In this approach, a manufacturer—say of cars, computers, or cameras—tries to produce as many items as possible just in time to deliver them to a buyer. Manufacturing a product and then having it sits in a warehouse, waiting to be sold; reduces profit. But manufacturers must balance certain variables: they must announce a price and stick to it, they must guess at how much delay or "lead time" they will need to deliver a product, and they must guess at how much demand for the product there will be. The goal, as always, is to earn maximum profit. It turns out that the solution of a cubic equation is central to solving the equation for maximizing profit (Chris Budd and Chris Sangwin).
HOSPITAL SIZE
Since the 1980s, hospitals have found it increasingly difficult to make a profit—or even to stay out of debt. Mathematical cost-profit analysis has therefore been brought into play to help hospitals make more profit. One basic decision that a hospital must make is how many beds to have. Having too few or too many beds makes it harder for a hospital to be profitable. Traditionally, profitability has been described as a quadratic function of bed size (the number of beds in the hospital, not how big each bed is); more recent work however has shown that a cubic equation works even better. (Other factors are involved, such as where the hospital is located and how affluent the surrounding population is. But if these assumptions are held steady, profitability is a cubic function of bed size.) Using a cubic equation, researchers have found that there isn't just one bed size that is most profitable, but two; or, rather, a point this is typical of a cubic equation, which can have two maximum points rather than one (as a quadratic equation does). From 0 to 238 beds, profit increases. After 238 beds it decreases until 560, after which it goes up indefinitely (but other factors prevent us from building infinitely large hospitals). A hospital is therefore most profitable, in the United States under current conditions, if it is either medium-sized (about 238 beds) or as big as it can be (560 beds or larger) ( Chris Budd and Chris Sangwin).
GUIDING WEAPONS
In steering weapons such as missiles and planes as in projectile motion, it is necessary to tell the computer that guides the weapon where it is. Each position is coded as a set of numbers, the "coordinates" of the weapon or vehicle. These can be given in traditional terms as latitude and longitude (numbers derived from a network of imaginary lines laid down on the Earth's surface by map-makers) plus altitude (height above the surface), or in terms of an "Earthcentered coordinate system." Since one type of coordinates is better for some purposes and the other is better for other purposes, it is sometimes necessary to translate between them—to take position information given in one form and turn it into the other form. Going from latitude-longitude coordinates to Earth-centered coordinates is mathematically easy, but going the other way requires the solution of a quartic equation (Chris Budd and Chris Sangwin)
APPLICATION IN CHEMICAL ANALYSIS OF ACID-BASE EQUILIBRIA AS IN DETERMINING THE PH OF A MONOPROTIC ACID SOLUTION:
A monoprotic acid is an acid having only one dissociable proton. Absorption of basic drugs was found to be a function of the whole solubility/pH relationship rather than a single solubility value at pH 7. The study of PH and its evaluation is therefore of relevance to life. In determining the PH of the monoprotic acid solution, strong acid approximation is known to assume a complete dissociation of the acid leading to a quadratic equation which must be solved to obtain the PH for a known analytical concentration of the acid. (Barry A. Hendriksen, Manuel V. Sanchez Felix, and Michael B. Bolger)
THE STUDY OF POPULATION
The quadratic equation X2 + X -1 = 0 arises in studies of the populations of rabbits and in the pattern in which the seeds of sunflowers and the leaves on the stems of plants are arranged. These are all linked with the Golden ratio through the Fibonacci sequence which is given by 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144,……………….. The aforementioned cubic and quartic equations that arose in the different areas of life may possibly be reduced to second order equations in which this algorithm can be applied.
REFERENCES
1. Chris Budd and Chris Sangwin, 101 uses of a quadratic equation Parts I and II at: http://plus.maths.org
2. Real life uses of quadratic equations at: http://mathforum.org
3. Olugbara, O.O., CUWPDB2005, Covenant University, Nigeria Algorithm design techniques
4. Barry A. Hendriksen, Manuel V. Sanchez Felix, and Michael B. Bolger; the Composite Solubility Versus pH Profile and Its Role in Intestinal Absorption Prediction.
Comments